Data - Formats
Class (Prototype) - Object (Instance)
A class (prototype) is a template that defines object properties and may also describe object behavior. An object is an instance of a class.
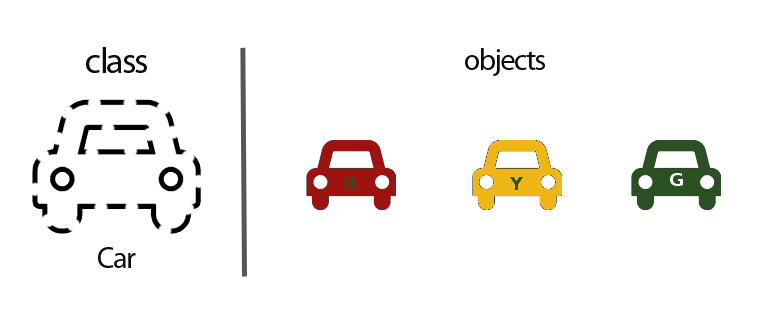
We can think of class as a sketch (prototype) of a car. It contains all the details about the number of tires, the doors, the color... Based on these descriptions we build a car, let’s say the Green car: this Green car is the object. As many cars can be made from the same prototype, we can create as many objects as we want from a class.
Find more details in the ‘Function - Class’ glossary.
Constant
A constant is a value or a variable with a value that will never change during the running time of the program. The keyword 'const' is used to define such data in most programming language:
const auto lastName = "CantBeChanged";
Data Structure
Data structures are the way we can store and retrieve data, they represent the knowledge to be organized in memory. No matter what problem are we solving, in one way or another we have to deal with them !
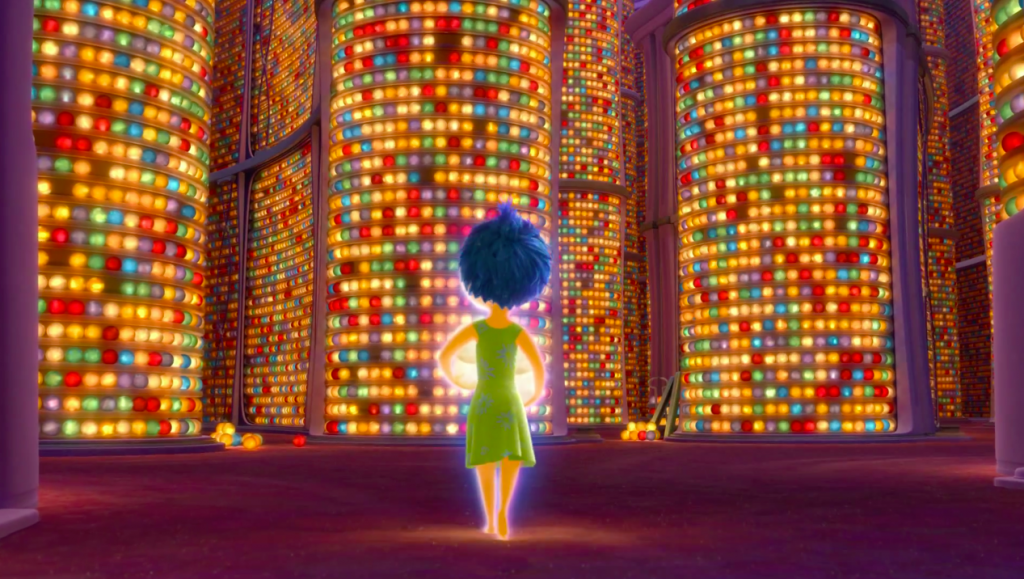
A data structure instance is an Object.
Null - Nullptr
Null and NullPtr represent an intentional absence of a value.
let uninstantiated = null; // JS
Car* lostPtr = nullptr; // C++
Pointer
A pointer is a variable that holds a memory address where a value lives. As an analogy, a page number in a book's index could be considered a pointer to the corresponding page.
Pointers are the foundation of how computers allocate and deal with memory in hardware. They allow us to read and write directly in memory space.
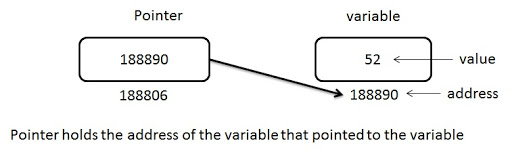
String
A string is a sequence of characters used to represent text.
const string = "hello world";A string can be manipulated as an Array containing chars (cf. below).
Primitives
The primitive data type is the basic building block of data. They hold one single value. They represent a char, number, or boolean.
Note: the actual range of primitive data types that is available is dependent upon the specific programming language that is being used (those are the most basic ones).
Boolean - bool
A boolean has 2 possible values: true or false.
bool truth = true;
Character - Char
It stores a single character and requires a single byte of memory:
const char letter = 'H';
Number - Integer (Int), Float...
A numeric data type.
const pi = 3.14; // :p
Undefined
Undefined with JS is a primitive value automatically assigned to a variable that has just been declared or to the argument of a function that has not been provided.
Formats
JSON
JSON stands for JavaScript Object Notation. It is a way to format data so that it can be human readable, storable as a string and thus be transmitted from one place to another. It is most commonly used in web development.
{ "id" : 8, "name" : "Globo", "functions" : ["solver", "player", "teacher"], "isAvailable" : true }
XML
XML stands for eXtensible Markup Language and was designed to store and transport data in a readable way for both humans and machines. Both XML and JSON have the same purpose.