TestMerge.cxx File Reference
#include <gtest/gtest.h>
#include <merge.hxx>
#include <functional>
#include <vector>
#include <string>
Include dependency graph for TestMerge.cxx:
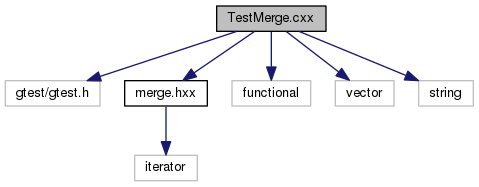
Go to the source code of this file.
Functions | |
TEST (TestMerge, MergeInPlaces) | |
TEST (TestMerge, MergeWithBuffers) | |
TEST (TestMerge, MergeSorts) | |
Function Documentation
TEST | ( | TestMerge | , |
MergeInPlaces | |||
) |
Definition at line 50 of file TestMerge.cxx.
Definition: merge.hxx:47
TEST | ( | TestMerge | , |
MergeWithBuffers | |||
) |
Definition at line 119 of file TestMerge.cxx.
160 MergeWithBuffer<IT>()(uniqueValueArray.begin(), uniqueValueArray.end(), uniqueValueArray.end());
169 MergeWithBuffer<IT>()(doubleValuesArray.begin(), doubleValuesArray.begin() + 1, doubleValuesArray.end());
177 std::string stringToMerge = "eknxasuw"; // Left sorted part [e,k,n,x] - Right sorted part [a,s,u,w]
Definition: merge.hxx:94
TEST | ( | TestMerge | , |
MergeSorts | |||
) |
Definition at line 188 of file TestMerge.cxx.