TestPrimsGen.cxx File Reference
Include dependency graph for TestPrimsGen.cxx:
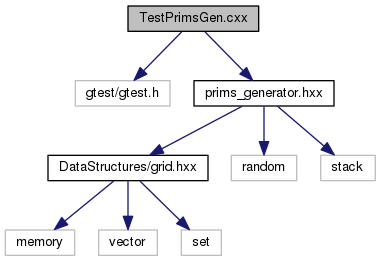
Go to the source code of this file.
Functions | |
TEST (TestPrimsGen, build) | |
Function Documentation
TEST | ( | TestPrimsGen | , |
build | |||
) |
Definition at line 33 of file TestPrimsGen.cxx.
Point struct is a convenient struct use to represent a 2D point for a grid (index position)...
Definition: grid.hxx:56
Definition: prims_generator.hxx:52